2 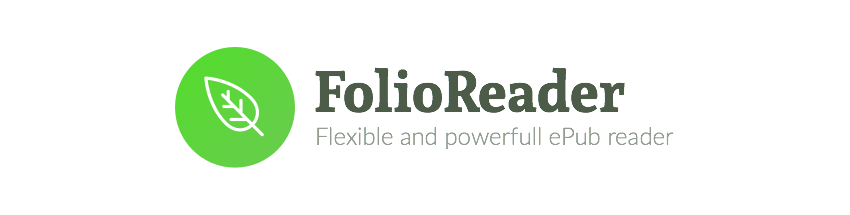
3 FolioReaderKit is an ePub reader and parser framework for iOS written in Swift.
5 
6 
7 
8 
12 - [x] ePub 2 and ePub 3 support
14 - [x] Custom Text Size
15 - [x] Text Highlighting
16 - [x] List / Edit / Delete Highlights
17 - [x] Themes / Day mode / Night mode
18 - [x] Handle Internal and External Links
19 - [x] Portrait / Landscape
20 - [x] Reading Time Left / Pages left
21 - [x] In-App Dictionary
22 - [x] Media Overlays (Sync text rendering with audio playback)
23 - [x] TTS - Text to Speech Support
24 - [x] Parse epub cover image
26 - [x] Vertical or/and Horizontal scrolling
27 - [x] Share Custom Image Quotes **<sup>NEW</sup>**
28 - [x] Support multiple instances at same time, like parallel reading **<sup>NEW</sup>**
30 - [ ] Add Notes to a Highlight
34 **Custom Fonts :smirk:** | **Text Highlighting :heart_eyes:**
35 :-------------------------:|:-------------------------:
36 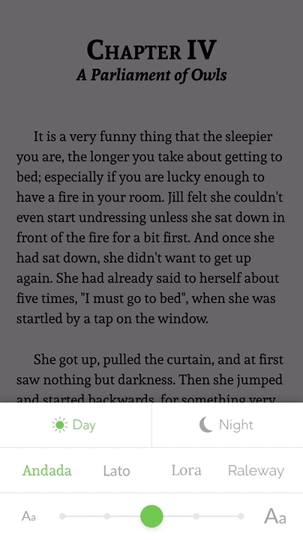 | 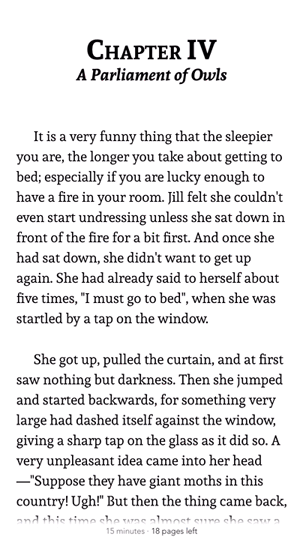
38 **Reading Time Left :open_mouth:** | **Media Overlays 😭**
39 :-------------------------:|:-------------------------:
40 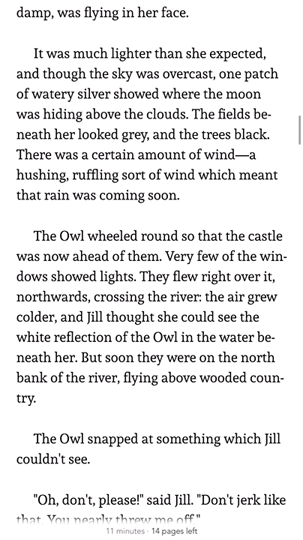 | 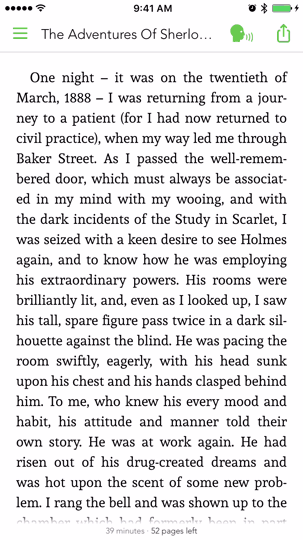
44 **FolioReaderKit** is available through [CocoaPods](http://cocoapods.org) and [Carthage](https://github.com/Carthage/Carthage).
48 [CocoaPods](http://cocoapods.org) is a dependency manager for Cocoa projects. You can install it with the following command:
51 $ gem install cocoapods
54 To integrate FolioReaderKit into your Xcode project using CocoaPods, specify it in your `Podfile`:
57 source 'https://github.com/CocoaPods/Specs.git'
61 target '<Your Target Name>' do
66 Then, run the following command:
72 Alternatively to give it a test run, run the command:
75 $ pod try FolioReaderKit
80 Add the following to your [Cartfile](https://github.com/Carthage/Carthage/blob/master/Documentation/Artifacts.md#cartfile)
83 github "FolioReader/FolioReaderKit"
86 Run the following command:
89 $ carthage update --platform iOS --no-use-binaries
92 Then, follow the steps as described in Carthage's [README](https://github.com/Carthage/Carthage#adding-frameworks-to-an-application).
101 To get started, this is a simple usage sample of using the integrated view controller.
104 import FolioReaderKit
106 func open(sender: AnyObject) {
107 let config = FolioReaderConfig()
108 let bookPath = Bundle.main.path(forResource: "book", ofType: "epub")
109 let folioReader = FolioReader()
110 folioReader.presentReader(parentViewController: self, withEpubPath: bookPath!, andConfig: config)
114 For more usage examples check the [Example](/Example) folder.
118 To get started, here is a simple example how to use the integrated view controller with storyboards.
121 import FolioReaderKit
123 class StoryboardFolioReaderContrainer: FolioReaderContainer {
124 required init?(coder aDecoder: NSCoder) {
125 super.init(coder: aDecoder)
127 let config = FolioReaderConfig()
128 config.scrollDirection = .horizontalWithVerticalContent
130 guard let bookPath = Bundle.main.path(forResource: "The Silver Chair", ofType: "epub") else { return }
131 setupConfig(config, epubPath: bookPath)
136 Go to your storyboard file, choose or create the view controller that should present the epub reader. In the identity inspector set StoryboardFolioReaderContrainer as class.
139 Checkout [Example](/Example) and [API Documentation](http://cocoadocs.org/docsets/FolioReaderKit)
141 You can always use the header-doc. (use **alt+click** in Xcode)
143 <img src="https://raw.githubusercontent.com/FolioReader/FolioReaderKit/assets/header-doc.png" width="521px"/>
146 If you are migrating to a newer version check out [MIGRATION](/MIGRATION.md) and [CHANGELOG](/CHANGELOG.md).
149 [**Heberti Almeida**](https://github.com/hebertialmeida)
151 - Follow me on **Twitter**: [**@hebertialmeida**](https://twitter.com/hebertialmeida)
152 - Contact me on **LinkedIn**: [**hebertialmeida**](http://linkedin.com/in/hebertialmeida)
155 FolioReaderKit is available under the BSD license. See the [LICENSE](/LICENSE) file.